15.13 Exercises
As you complete these exercises, you may find the PySimpleGUI documentation helpful, especially the call reference.
The exercises below are hosted on Trinket.io. Note that you need to have a Trinket.io account in order to submit your work. Follow these steps to submit your work:
-
Make sure you are signed into your Trinket.io account.
Go to Trinket.io to log in. Then close that browser tab and refresh this page. -
Remix the code to save a copy to your account.
- Work on your code.
-
Click on the Share button and choose Link.
- Add the link to your Assignments spreadsheet in your Google shared folder. Refer to the Instructions page for details on how to use the spreadsheet.
Exercise 15.1
Remembering that window layout is just a list of lists, with each inner list representing a row of the resulting window, produce this output in the trinket below. The first row is a text element, and the second is a button element.
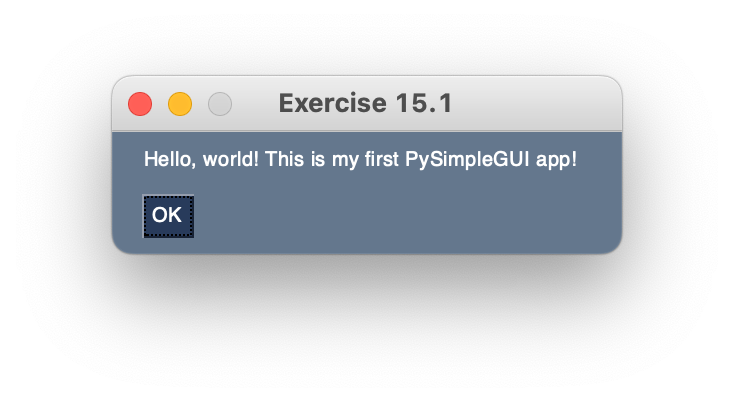
Exercise 15.2
The window in Exercise 15.1 closed immediately upon clicking the OK button. This behavior is similar to how an
sg.popup()
element works. Therefore, sg.popup()
would usually be preferred for this
type of behavior.
For more interactive windows, an event loop is used. The following trinket is stubbed out with an event loop.
When 'reading' a window, the Window.read()
function returns a tuple consisting of the
event
that occurred (e.g. button presses) and user input values
. The event loop is
where the app's behavior is coded.
TODO
Complete the layout to replicate the following app window. The first row consists of sg.Text()
and sg.InputText()
elements. The size for the input field is (15, 1)
. The second row
is two sg.Button()
elements.
Write if
statements to test which button was pressed. Use sg.popup()
statements
(instead of print
) to indicate what event occurred. In the case of the OK button, output the name
that was entered. See the popup examples here for the desired output. Note that the event corresponding to
closing the window has been coded for you. Note: Glielmo is just the name I entered for testing.
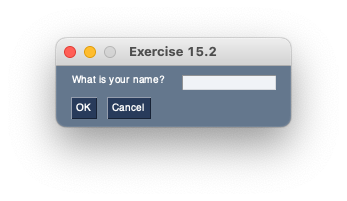
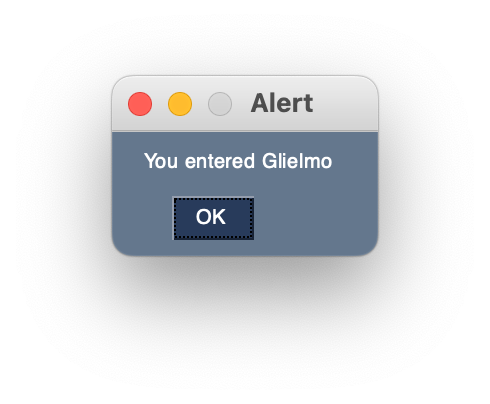
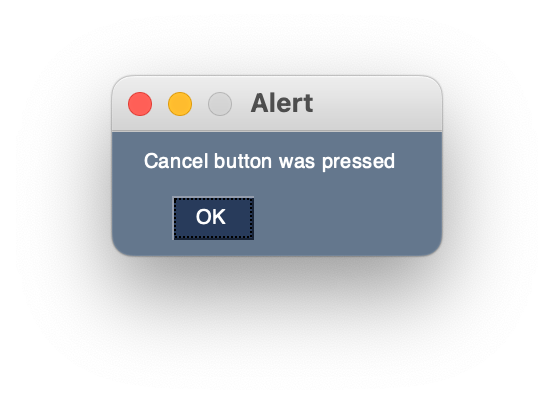
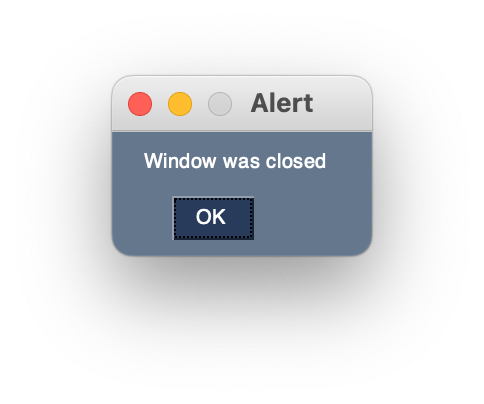
Exercise 15.3
In this exercise, we will add sg.InputText()
, sg.Radio()
, and
sg.Multiline()
elements. Refer to the PySimpleGUI documentation for
The Elements
for details on those elements.
We will focus on correct layout for this exercise. In the next exercise, we will add actions.
Details: Width for the labels on the left is 10. They are right-justified. The text input field has a
width of 20. sg.VPush()
is used to provide vertical separation where needed. Elements that get user
input need to have a key=
set. All radio buttons in a group need to have the same
groupid
. Size for the sg.Multiline()
element is (35, 8)
.
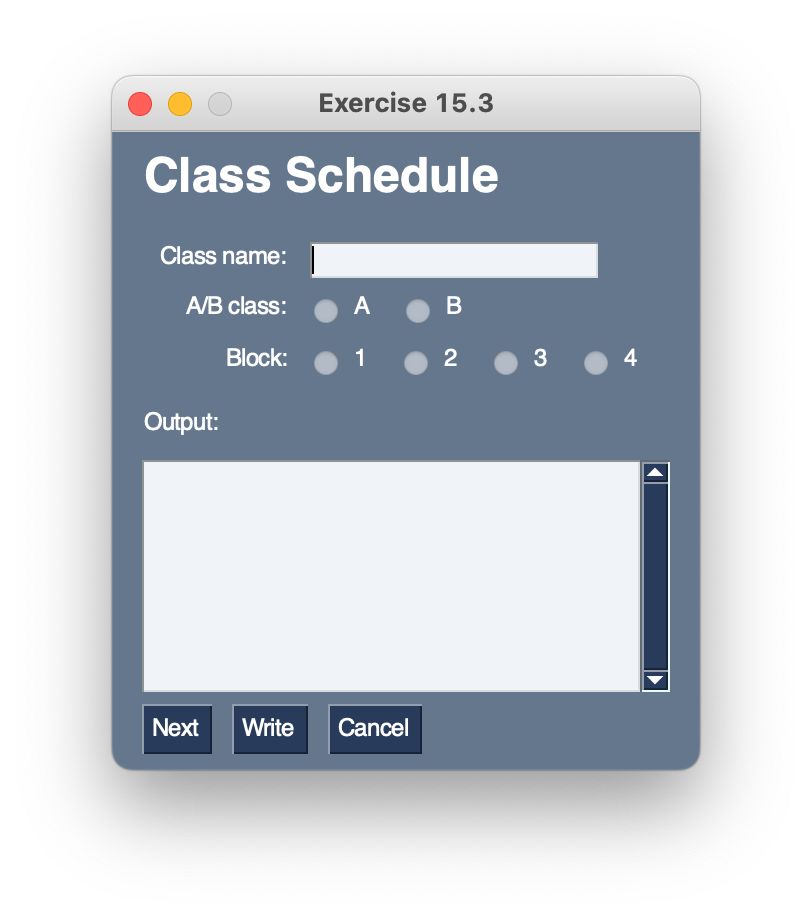
Exercise 15.4
Now it's time to add the actions. In the event loop, test for each button press. Code the appropriate actions for each.
-
Buttons
- Next
- If a course name has been entered, comma-separated values are added to the
output
variable for the course name, day, and block. All values are surrounded by double quotes. The Multiline element is updated withoutput
. The radio buttons are reset (toFalse
). Focus is set to the InputText element.
- If a course name has been entered, comma-separated values are added to the
- Write
- If course(s) have been entered, using a context manager, write
output
tooutput.csv
. Display a popup stating that the class schedule has been written to the file. Break out of the event loop.
- If course(s) have been entered, using a context manager, write
- Cancel
- Already coded.
- Next
-
Layout
- Clear the Input element (see docs).
- Clear the Multiline element and set it to automatically refresh (see docs).
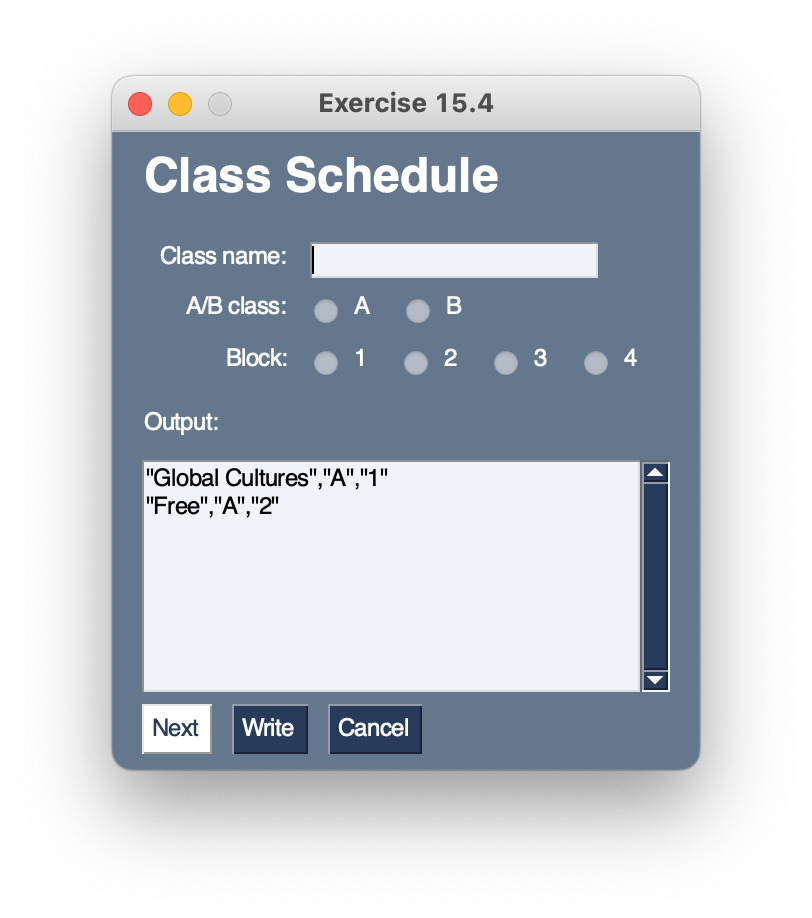
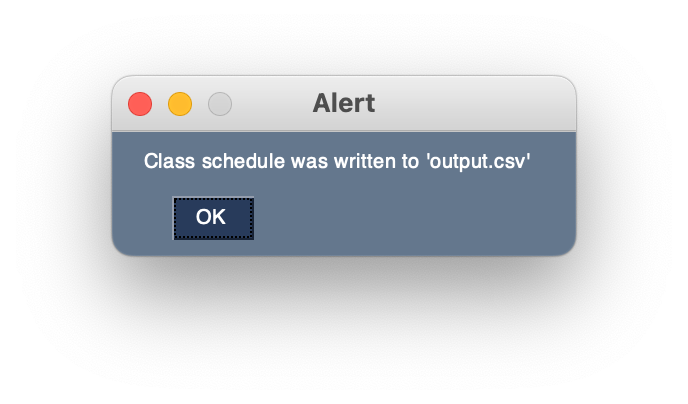