15.4 PySimpleGUI Layout
Your window's layout is a "list of lists" (LOL). Windows are broken down into "rows". Each row in your window becomes a list in your layout. Concatenate together all of the lists and you've got a layout...a list of lists.
Here is a layout to ask the user for some information.
import PySimpleGUI as sg
layout = [ [sg.Text("What's your name?")], # row 1
[sg.Input()], # row 2
[sg.Button('Ok')] ] # row 3
Notice that layout
is a list in which each element is also a list (representing a row in the
layout). The elements needed on each row are members of the inner lists.
Simple, as well as complex layouts can be achieved with this method. No cumbersome layout managers required.
Adding this layout to a window is simple.
window = sg.Window('Input Window', layout)
event, values = window.read()
Here is the window produced by that code:
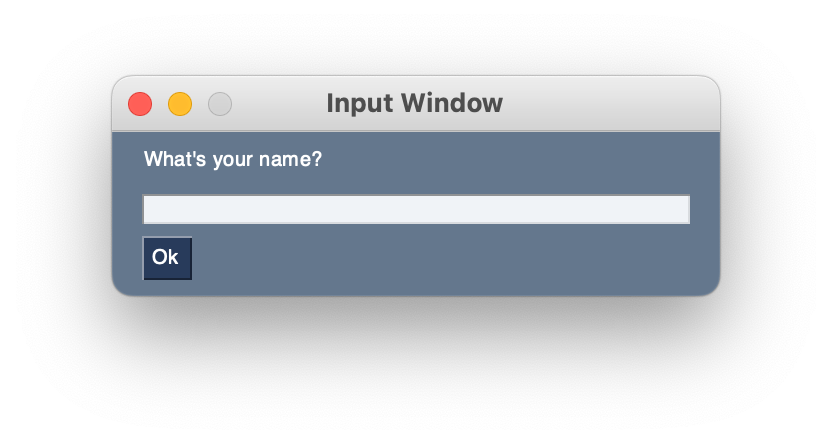
Notice that the three rows in the window correspond to the three inner lists of layout
.