15.5 Element Groupings
You will design a more user-friendly interface if you group and organize your elements in a coherent design. PySimpleGUI has several basic ways to group widgets. These are described in the following table. They are often referred to as “container” elements because in the element hierarchy of a GUI program they are the parent element of a group of related elements.
Element | Purpose |
---|---|
Frame | A Frame Element that contains other Elements. Encloses with a line around elements and a text label. |
TabGroup | TabGroup Element groups together your tabs into the group of tabs you see displayed in your window. |
Tab | Tab Element is another "Container" element that holds a layout and displays a tab with text. Used with TabGroup only Tabs are never placed directly into a layout. They are always "Contained" in a TabGroup layout. |
Pane | A sliding Pane that is unique to tkinter. Uses Columns to create individual panes. |
Column | A container element that is used to create a layout within your window's layout. |
Here is an example of PySimpleGUI groupings (containers):
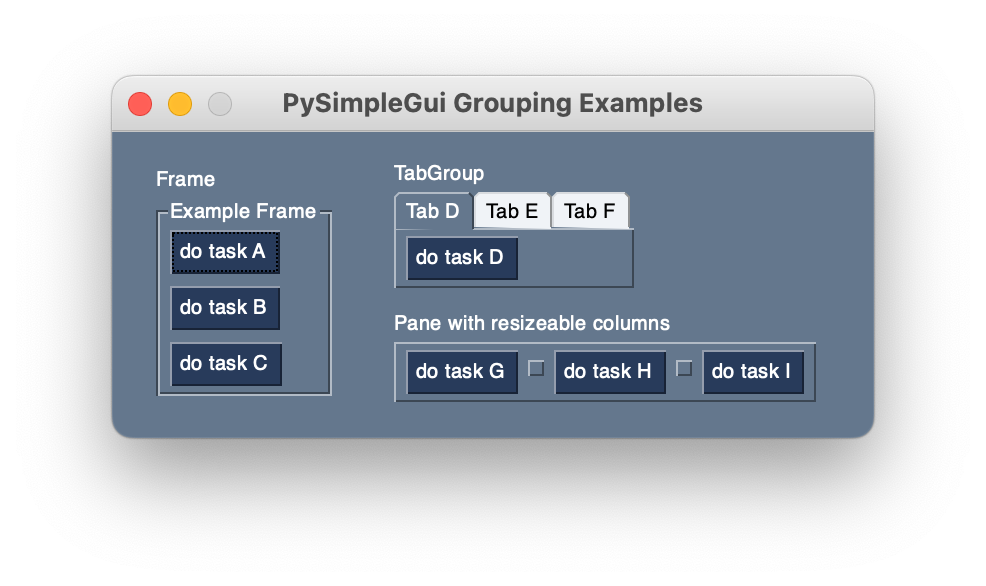
And here is the code:
import PySimpleGUI as sg
frame = sg.Frame('Frame', [
[sg.Frame('Example Frame', [[sg.Button('do task A')], [sg.Button('do task B')], [sg.Button('do task C')]])]],
relief=sg.RELIEF_FLAT)
tabgroup = sg.Frame('TabGroup', [[sg.TabGroup([[sg.Tab('Tab D', [[sg.Button('do task D')]]),
sg.Tab('Tab E', [[sg.Button('do task E')]]),
sg.Tab('Tab F', [[sg.Button('do task F')]])]])]], relief=sg.RELIEF_FLAT)
pane = sg.Frame('Pane with resizeable columns',
[[sg.Pane([sg.Column([[sg.Button('do task G')]]), sg.Column([[sg.Button('do task H')]]),
sg.Column([[sg.Button('do task I')]])],
orientation='horizontal')]], relief=sg.RELIEF_FLAT)
layout = [
[frame, sg.Frame('', [[tabgroup], [pane]], relief=sg.RELIEF_FLAT)],
]
window = sg.Window('PySimpleGUI Grouping Examples', layout)
event, values = window.read()
layout
is a list of lists with only one row (inner list). That row contains two elements: frame
and a sg.Frame
element containing tabgroup
and pane
stacked vertically.
sg.Window
takes layout
as an argument to create the window.